Arduino interfacing stepper motor with LCD in proteus
In this article we will learn how to interfacing arduino wit stepper motor and LCD in proteus.
In the last post we will learn how to interfacing arduino with HX711 and load cell. You can visit our website,
I hope you appreciate my work, let’s discuss about today’s project.
Diagram of this project:
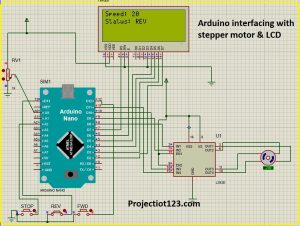
Components which we use in this project:
- Arduino nano
- Stepper motor
- Liquid crystal display (LCD)
- L293D driver stepper motor
- Push button (3)
- Potentio meter
- Connecting wires
Construction of arduino interfacing with stepper motor with LCD in proteus:
- Connect RS point of LCD to D7 point of arduino nano.
- Connect LCD point EN to D6 point of arduino nano.
- Connect D4 point of LCD to D5 point of arduino nano.
- Connect D5 point of LCD to D4 point of arduino nano.
- Connect D6 point of LCD to D3 point of arduino nano.
- Connect D7 point of LCD to D2 point of arduino nano.
- Connect K point of LCD to GND point of arduino nano.
- Connect VDD point of LCD to 5V point of arduino.
- Connect VO point LCD to GND point of arduino.
- Connect the control pins of the driver circuit to appropriate pins on the arduino like (motor control pins and step/dir pins)
Working of arduino interfacing with stepper motor with LCD.
The working of a stepper motor with an LCD involves integrating the two components to create a system where the stepper motor’s movement is controlled and displayed on the LCD screen
Application of arduino interfacing with stepper motor with LCD.
Stepper motor combined with LCD display find application in various fields where accurate positioning and visual feedback are crucial,
Here are some examples of how this combination can be used:
- CNC machines and 3D printers
- Automated manufacturing system
- Scientific instruments
- Medical devices
- Automotive
- Textile machinery
- Industrial automation
Advantages of arduino interfacing with stepper motor with LCD.
Combining a stepper motor with LCD offers several advantages which are listed below.
- Accurate positioning
- Real-time feedback
- User interface
- Visualization
- Process monitoring
Program coding of arduino interfacing with stepper motor with LCD.
#include <Stepper.h>
#include <LiquidCrystal.h>
LiquidCrystal lcd(7, 6, 5, 4, 3, 2);
#define STEPS 100
Stepper stepper(STEPS, 8, 9, 10, 11);
int previous = 0;
int motorSpeed = 20; // Initial motor speed
const int forwardButtonPin = 12; // Pin for the forward button
const int reverseButtonPin = 13; // Pin for the reverse button
const int stopButtonPin = 2; // Pin for the stop button
void setup() {
stepper.setSpeed(motorSpeed);
lcd.begin(16, 2);
lcd.print(“Speed:”);
lcd.setCursor(7, 0);
lcd.print(motorSpeed); // Display initial speed value
lcd.setCursor(0, 1);
lcd.print(“Status: STOP”); // Display initial status
pinMode(forwardButtonPin, INPUT_PULLUP);
pinMode(reverseButtonPin, INPUT_PULLUP);
pinMode(stopButtonPin, INPUT_PULLUP);
}
void loop() {
int val = analogRead(A0);
int numSteps = map(val, 0, 1023, 0, 200); // Adjust the range of steps as needed
// Read button states
int forwardButtonState = digitalRead(forwardButtonPin);
int reverseButtonState = digitalRead(reverseButtonPin);
int stopButtonState = digitalRead(stopButtonPin);
// Move the motor based on button presses and update status
if (forwardButtonState == LOW) {
stepper.step(numSteps);
lcd.clear();
lcd.print(“Speed:”);
lcd.setCursor(0, 1);
lcd.print(“Status:”);
lcd.setCursor(8, 1);
lcd.print(“FWD “);
}
if (reverseButtonState == LOW) {
stepper.step(-numSteps);
lcd.clear();
lcd.print(“Speed:”);
lcd.setCursor(0, 1);
lcd.print(“Status:”);
lcd.setCursor(8, 1);
lcd.print(“REV “);
}
if (stopButtonState == 0) {
stepper.step(0); // Stop the motor
lcd.clear();
lcd.print(“Speed:”);
lcd.setCursor(0, 1);
lcd.print(“Status:”);
lcd.setCursor(8, 1);
lcd.print(“STOP”);
}
// Display the current speed on the LCD
lcd.setCursor(7, 0);
lcd.print(” “); // Clear previous value
lcd.setCursor(7, 0);
lcd.print(motorSpeed);
// You can add a delay here if needed
delay(100);
}