
DIY Ultrasonic Range Finder with ESP32
In this Article, we learn about how to make DIY Ultrasonic Range Finder with ESP32.
In the Last Post, we learned about How to make OR Gate Circuit in proteus.
I hope you appreciate my work, Let’s Discuss about Today project.
Components required:
- ESP32 board
- Sonar sensor
- Power supply
- Jumper wires
Circuit Diagram:
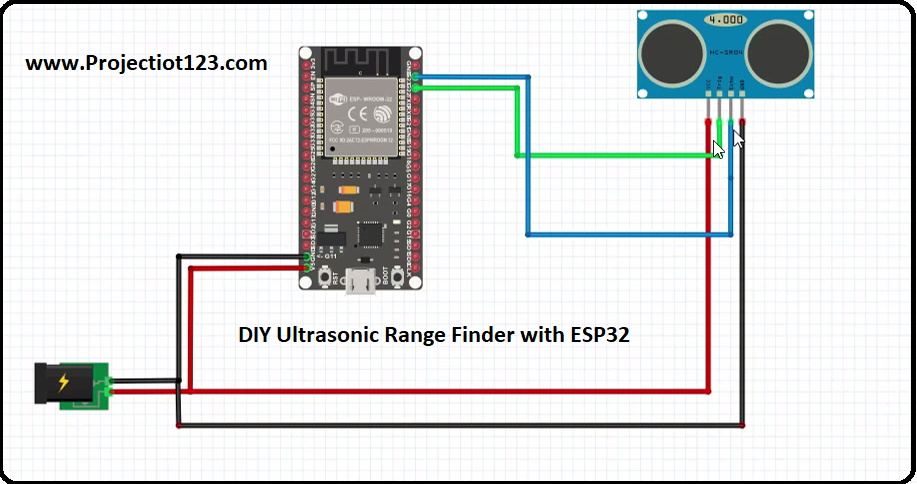
Hardware:
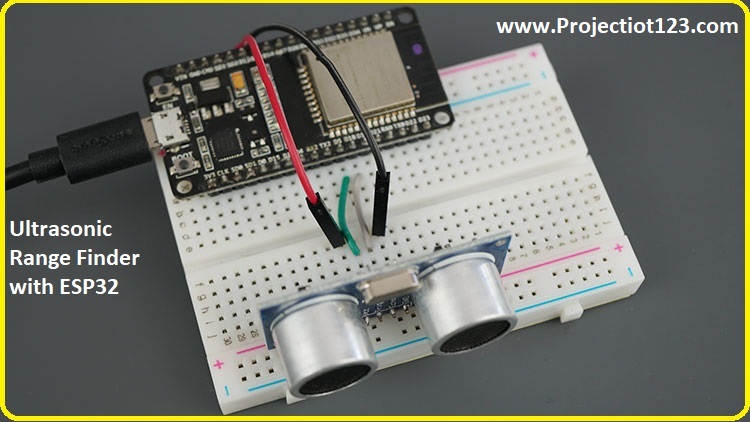
Working Overview:
An ultrasonic range finder can be connected to an ESP32 microcontroller to measure distances using sound waves. The ESP32 has GPIO pins that can be used to interface with the ultrasonic sensor.
Connect the trigger pin of the ultrasonic sensor to a GPIO pin on the ESP32, and the echo pin to another GPIO pin. Then, you can use a simple code to trigger the sensor, measure the time it takes for the sound wave to bounce back, and calculate the distance based on the speed of sound.


Usages:
- Distance Measurement
- Gesture Recognition
- Parking Assistance
- Liquid Level Measurement
- IoT Applications
Programming Code:
[dt_code]
#include <ESPAsyncWebServer.h>
#include <WiFi.h>
#include <Wire.h>
const char *ssid = “Microsolutions”; // Your WiFi SSID
const char *password = “@1yahoo.com”; // Your WiFi password
// HC-SR04 Ultrasonic Sensor Pins
const int trigPin = 22;
const int echoPin = 23;
// Define sound speed in cm/uS
#define SOUND_SPEED 0.034
#define CM_TO_INCH 0.393701
long duration;
float distanceCm;
float distanceInch;
// Create an instance of the server
AsyncWebServer server(80);
void setup() {
Serial.begin(115200); // Starts the serial communication
// Connect to Wi-Fi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println(“Connecting to WiFi…”);
}
Serial.println(“Connected to WiFi”);
Serial.print(“IP Address: “);
Serial.println(WiFi.localIP());
pinMode(trigPin, OUTPUT); // Sets the trigPin as an Output
pinMode(echoPin, INPUT); // Sets the echoPin as an Input
// Serve HTML page for distance display
server.on(“/”, HTTP_GET, [](AsyncWebServerRequest *request) {
String html = “<html><head>”;
html += “<link href=’https://fonts.googleapis.com/css2?family=Roboto:wght@300;400&display=swap’ rel=’stylesheet’>”;
html += “<script src=’https://cdn.jsdelivr.net/npm/justgage’></script>”;
html += “<script src=’https://cdn.jsdelivr.net/npm/raphael@2.2.8′></script>”;
html += “<style>”;
html += “body { font-family: ‘Roboto’, sans-serif; text-align: center; background-color: #f0f0f0; }”;
html += “.container { max-width: 600px; margin: 0 auto; padding: 20px; }”;
html += “h1 { color: #333; }”;
html += “p { font-size: 24px; color: #555; }”;
html += “</style>”;
html += “<script type=’text/javascript’>”;
html += “function updateDistance() {“;
html += ” var xhr = new XMLHttpRequest();”;
html += ” xhr.onreadystatechange = function() {“;
html += ” if (xhr.readyState == 4 && xhr.status == 200) {“;
html += ” var distanceData = JSON.parse(xhr.responseText);”;
html += ” document.getElementById(‘distanceCm’).innerHTML = ‘Distance (cm): ‘ + distanceData.cm;”;
html += ” document.getElementById(‘distanceInch’).innerHTML = ‘Distance (inch): ‘ + distanceData.inch;”;
html += ” g.refresh(distanceData.cm);”; // Update the gauge with the distance in cm
html += ” }”;
html += ” };”;
html += ” xhr.open(‘GET’, ‘/getDistance’, true);”;
html += ” xhr.send();”;
html += “}”;
html += “setInterval(updateDistance, 1000);”; // Update distance every second
html += “</script>”;
html += “</head><body>”;
html += “<div class=’container’>”;
html += “<h1>Distance Measurement</h1>”;
html += “<p id=’distanceCm’>Distance (cm): Loading…</p>”;
html += “<p id=’distanceInch’>Distance (inch): Loading…</p>”;
html += “<div id=’gauge’ style=’width:300px; height:250px; margin: 0 auto;’></div>”;
html += “</div>”;
html += “<script>”;
html += “var g = new JustGage({“;
html += ” id: ‘gauge’,”;
html += ” value: 0,”;
html += ” min: 0,”;
html += ” max: 200,”;
html += ” title: ‘Distance (cm)’,”;
html += ” label: ‘cm’,”;
html += ” levelColors: [‘#00FF00’, ‘#FFD700’, ‘#FF0000’]”;
html += “});”;
html += “</script>”;
html += “</body></html>”;
request->send(200, “text/html”, html);
});
// Route to get the current distance in JSON format
server.on(“/getDistance”, HTTP_GET, [](AsyncWebServerRequest *request) {
String distanceData = “{\”cm\”:” + String(distanceCm) + “, \”inch\”:” + String(distanceInch) + “}”;
request->send(200, “application/json”, distanceData);
});
// Start server
server.begin();
}
void loop() {
// Ultrasonic sensor code
digitalWrite(trigPin, LOW);
delayMicroseconds(2);
digitalWrite(trigPin, HIGH);
delayMicroseconds(10);
digitalWrite(trigPin, LOW);
duration = pulseIn(echoPin, HIGH);
distanceCm = duration * SOUND_SPEED / 2;
distanceInch = distanceCm * CM_TO_INCH;
// Print distance from ultrasonic sensor
Serial.print(“Distance (cm): “);
Serial.println(distanceCm);
Serial.print(“Distance (inch): “);
Serial.println(distanceInch);
delay(1000); // Delay between distance measurements
}
[/dt_code]